简介
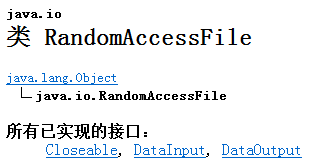
- 其父类为Object,并没有继承字符流或字节流的任一个类
- 实现了DataInput、DataOutput接口,意味着这个类即可读又可写。
- 是io体系中功能最丰富的文件访问类,它提供了众多的方法来访问文件内容
- 可以通过设置游标来自由访问文件的任意位置,使用于访问文件部分内容的情况。
- 可以用来访问保存数据记录的文件,但是必须知道这些数据的类型和位置
写入文件
保存一个类的各个域到文件
public class RandomAccessFileTest { class Person { int id; String name; double height; public Person(){ } public Person(int id, String name, double height) { this.id = id; this.name = name; this.height = height; } //把属性写进去 public void write(RandomAccessFile raf) throws IOException { raf.write(id); //int型的怎么写都不会出错了 raf.writeUTF(name); //可能含中文,指定下编码,不过写到文件里打开看可能还是会乱码,因为这个只能保证读回来的时候不乱 raf.writeDouble(height); } } public static void main(String[] args) throws IOException { RandomAccessFile raf = new RandomAccessFile("d:/data.txt","rw"); Person p = new Person(1001,"xiaoming",1.80d); p.write(raf); } }
- 指定方式打开
读取文件
我们把刚才那个文件读回来,先给Person类加个read方法。注意:读取的时候一定要严格按照写入的顺序,用相应的数据类型读取方式来读
public void read(RandomAccessFile raf) throws IOException { this.id = raf.readInt(); this.name = raf.readUTF(); this.height = raf.readDouble(); }
注意,读的时候保证指针在开头
public static void main(String[] args) throws IOException { RandomAccessFile raf = new RandomAccessFile("d:/data.txt", "rw"); Person p = new Person(1001, "xiaoming", 1.80d); p.write(raf);// 写入文件后,任意访问文件的指针在文件的结尾 raf.seek(0);// 读取时,将指针重置到文件的开始位置。 Person p2 = new Person(); //等着接收文件内容 p2.read(raf); System.out.println("id=" + p2.getId() + ";name=" + p2.getName() + ";height=" + p2.getHeight()); }
结果
id=1001;name=xiaoming;height=1.8
追加内容
直接把游标设置到末尾就可以了
public static void main(String[] args) throws IOException { RandomAccessFile raf = new RandomAccessFile("D:/out.txt","rw"); raf.seek(raf.length()); raf.write("\r\n中国移动阅读基地".getBytes()); }
- 结果
插入内容
插入的话需要先把目标位置后面的内容读入缓存文件,然后追加新内容,最后把原内容写回来
private static void insert(String fileName,long pos,String content) throws IOException {
//创建临时空文件 File tempFile = File.createTempFile("temp",null); //在虚拟机终止时,请求删除此抽象路径名表示的文件或目录 tempFile.deleteOnExit(); FileOutputStream fos = new FileOutputStream(tempFile); RandomAccessFile raf = new RandomAccessFile(fileName,"rw"); raf.seek(pos); byte[] buffer = new byte[4]; int num = 0; while(-1 != (num = raf.read(buffer))) { fos.write(buffer,0,num); } raf.seek(pos); raf.write(content.getBytes()); FileInputStream fis = new FileInputStream(tempFile); while(-1 != (num = fis.read(buffer))) { raf.write(buffer,0,num); }
}